Table of Contents
PHP Data Objects (PDO) is an extension that provides a lightweight, consistent, and secure interface for connecting to databases such as MySQL, PostgreSQL, and SQLite. PHP Data Objects uses prepared statements, which help prevent SQL injection attacks and improve performance. In this article, we will discuss how to connect to a MySQL database using PHP Data Objects and explain the code and its functions.
PHP, or Hypertext Preprocessor, is a widely-used open-source scripting language designed for web development. It allows developers to create dynamic and interactive websites by embedding server-side code into HTML. One of the most common tasks in web development is connecting to a database to store, retrieve, or manipulate data.
Advantages of using PHP Data Objects
There are several advantages to using PHP Data Objects for database interactions in your web development projects:
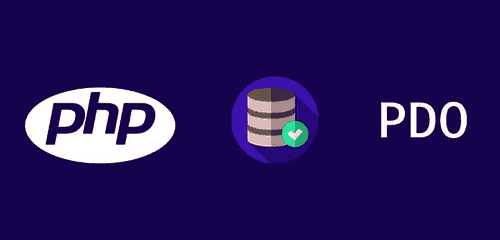
- Consistent Interface: PHP Data Objects provides a consistent and unified API for working with different databases such as MySQL, PostgreSQL, and SQLite. This consistency makes it easier to switch between database systems without having to rewrite much of your code.
- Prepared Statements: PHP Data Objects supports prepared statements, which help prevent SQL injection attacks. By separating SQL queries from user input, prepared remarks to ensure that user data is not interpreted as SQL code, thus reducing the risk of malicious attacks.
- Performance: Prepared statements can improve performance when executing the same query multiple times with different parameters. Since the database server only needs to parse and optimize the query once, it can save time and resources.
- Error Handling: PHP Data Objects allows for flexible error handling through different error modes, such as exceptions or warnings. By setting the error mode to PDO::ERRMODE_EXCEPTION, developers can handle errors more effectively using try-catch blocks.
- Transaction Support: PHP Data Objects supports transactions, which enable you to group multiple database operations into a single unit of work. This can help ensure data consistency and integrity by allowing you to roll back changes if an error occurs.
- Fetch Modes: PHP Data Objects provides several fetch modes that allow you to control how data is retrieved from the database. For example, you can fetch data as associative arrays or objects or even bind the results to specific variables, giving you more control over your application’s data handling.
- Portability: Since PHP Data Objects is a native extension of PHP, it is widely supported across different platforms and operating systems. This makes it easier to deploy and maintain your applications in various environments.
By leveraging the benefits of PHP Data Objects, developers can create more secure, efficient, and maintainable web applications that effectively handle database interactions.
PHP Data Objects Database Connection Code
<?php
// Database configuration
$db_host = "localhost";
$db_name = "your_database_name";
$db_username = "your_username";
$db_password = "your_password";
try {
// Create a new PDO instance
$pdo = new PDO("mysql:host=$db_host;dbname=$db_name", $db_username, $db_password);
// Set the PDO error mode to exception
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
echo "Connected successfully";
} catch (PDOException $e) {
echo "Connection failed: " . $e->getMessage();
}
?>
Establishing a MySQL Database Connection Using PHP Data Objects
- $db_host: This variable holds the hostname of your database server. This will usually be “localhost” if the database is on the same server as your PHP application.
- $db_name: This variable holds the database name you want to connect to.
- $db_username: This variable holds the username you use to authenticate with the database.
- $db_password: This variable holds the password associated with the database username.
- Try and catch block: This part of the code attempts to create a new PDO instance and connect to the database. If any error occurs during the Connection, the catch block will handle the error and display a message.
- $pdo: This variable holds the new PDO instance. PDO (PHP Data Objects) is a PHP extension providing a consistent interface for databases. It supports prepared statements, which can help prevent SQL injection attacks.
- PDO::ATTR_ERRMODE: This attribute sets the error reporting mode for the PDO instance. In this case, we set it to PDO::ERRMODE_EXCEPTION, which means that if an error occurs, an exception will be thrown.
- Echo “Connected successfully”: This message will be displayed if the Connection is successful.
- PDOException $e: If any exception occurs during the connection process, this variable will hold the details of the exception.
- Echo “Connection failed: ” . $e->getMessage(): This line displays the error message if the Connection fails.
This code demonstrates how to connect to a MySQL database using PHP Data Objects. You can customize the variables at the script’s beginning to match your database configuration.
In conclusion, using PHP PDO to establish a connection with a MySQL database is an efficient and secure method for web development projects. PDO significantly enhances the security and performance of database interactions with its consistent interface, support for various databases, and built-in features such as prepared statements.
By understanding the different components of the PHP Data Objects connection code, developers can create customizable and maintainable applications that effectively interact with databases. As web development continues to evolve, utilizing best practices like PHP PDO ensures that your projects remain robust and adaptable in the face of new technologies and security threats.