Table of Contents
Web authentication is an essential aspect of building secure web applications or websites. A login form is a crucial feature that allows users to authenticate themselves by entering their credentials, such as a username and password, to access restricted areas or personalized content.
In PHP, creating a login form involves creating an HTML form that collects user credentials and sending that data to a PHP script that verifies the user’s input and sets up a user session. This article will explore how to create a login form and the PHP script that handles the login process.
How to Create a MySQL Database and Insert Data using PHP
You can easily create a MySQL database and insert data using the mysqli extension. First, you need to establish a connection to the database and then create a table to hold the data. Once the table is created, you can use SQL statements to insert data into the table. You can manage and store data in a MySQL database using PHP.
<?php
// Information for database connection
$servername = "localhost";
$username = "your_username";
$password = "your_password";
$dbname = "your_database";
// Creating the database connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Checking for connection errors
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// Creating the users table
$sql = "CREATE TABLE users (
id INT NOT NULL AUTO_INCREMENT,
username VARCHAR(50) NOT NULL,
password VARCHAR(50) NOT NULL,
PRIMARY KEY (id)
)";
if ($conn->query($sql) === TRUE) {
echo "Table users created successfully<br>";
} else {
echo "Error creating table: " . $conn->error . "<br>";
}
// Adding a user
$username = "admin";
$password = "mypassword";
$sql = "INSERT INTO users (username, password) VALUES ('$username', '$password')";
if ($conn->query($sql) === TRUE) {
echo "New user created successfully";
} else {
echo "Error: " . $sql . "<br>" . $conn->error;
}
//Closing the final database connection
$conn->close();
?>
Web Authentication
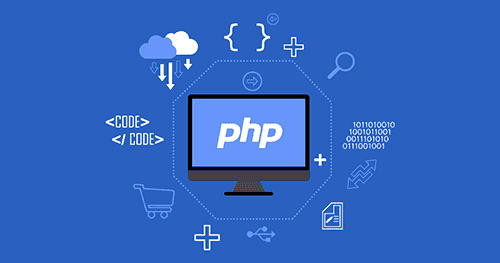
Login Form – Web Authentication
<div class="container">
<?php
session_start();
if (isset($_SESSION['username'])) {
echo '<h2>Dashboard</h2>';
echo '<p class="warning">You are already logged in as ' . $_SESSION['username'] . '.</p>';
echo '<a class="logout" href="logout.php">Logout</a>';
} else {
echo '<h2>Login</h2>';
if (isset($_SESSION['login_error'])) {
echo '<p class="warning">' . $_SESSION['login_error'] . '</p>';
unset($_SESSION['login_error']);
}
echo '<form action="login.php" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
<input type="submit" value="Login">
</form>';
}
echo '<p><a href="dashboard.php">Go to the dashboard page</a></p>';
?>
</div>
CSS
body {
font-family: Arial, sans-serif;
background-color: #f2f2f2;
margin: 0;
padding: 0;
}
.container {
background-color: #fff;
border-radius: 5px;
box-shadow: 0 0 10px rgba(0,0,0,0.3);
margin: 50px auto;
max-width: 400px;
padding: 20px;
}
h2 {
text-align: center;
}
label {
display: block;
margin-bottom: 10px;
font-weight: bold;
}
input[type="text"], input[type="password"] {
padding: 10px;
margin-bottom: 20px;
width: 100%;
border-radius: 3px;
border: 1px solid #ccc;
box-sizing: border-box;
}
input[type="submit"] {
background-color: #4CAF50;
color: #fff;
padding: 10px;
border: none;
border-radius: 3px;
cursor: pointer;
width: 100%;
}
.alert {
background-color: #f44336;
color: white;
padding: 10px;
margin-bottom: 20px;
border-radius: 3px;
display: <?php echo isset($_SESSION['username']) ? "none" : "block"; ?>;
}
.logout {
background-color: #4CAF50;
color: #fff;
padding: 10px;
border: none;
border-radius: 3px;
cursor: pointer;
width: 100%;
display: <?php echo isset($_SESSION['username']) ? "block" : "none"; ?>;
}
.container p.warning {
background-color: #ffc107;
color: #222;
padding: 10px;
border-radius: 5px;
margin-bottom: 10px;
}
.container a.logout {
background-color: #dc3545;
color: #fff;
padding: 5px;
border-radius: 5px;
text-decoration: none;
display: inline-block;
}
.container h2 {
display: <?php echo isset($_SESSION['username']) ? 'block' : 'none'; ?>;
}
How to Set the Title of an HTML Page in PHP Based on User Login Status – Web Authentication
This code sets the title of the HTML page depending on the user’s login status.
The code first starts a session using the session_start() function. Then, it checks if the session variable ‘username’ is set using the isset() function. If the ‘username’ session variable is set, it means the user is already logged in, and the code sets the HTML page’s title to “Already Logged” by using the echo statement to output the title tag with the title text.
On the other hand, if the ‘username’ session variable is not set, it means the user is not logged in, and the code sets the HTML page’s title to “Login” by using the echo statement to output the title tag with the title text.
Setting the title of an HTML page is crucial as it helps users understand the page’s content and helps search engines index the page’s content correctly. By changing the page title depending on the user’s login status, the website can provide relevant information and improve the user experience.
<?php
session_start();
if (isset($_SESSION['username'])) {
echo '<title>Already Logged</title>';
} else {
echo '<title>Login</title>';
}
?>
Creating a MySQL Database Connection and Error Handling in PHP’s MySQLi Extension – Web Authentication
This code establishes a connection to a MySQL database using PHP’s MySQLi (MySQL Improved) extension. The code creates four variables to store the database server name, username, password, and the database name to be connected.
Then, the mysqli() function is called to create a new MySQLi object, which is used to interact with the MySQL database. The mysqli() function takes the server name, username, password, and database name as parameters to establish the connection.
After establishing the connection, the code checks for any connection errors using the connect_error property of the MySQLi object. If there is a connection error, the die() function is called, which terminates the PHP script and outputs an error message indicating the cause of the connection error.
In summary, this code establishes a connection to a MySQL database using PHP’s MySQLi extension and checks for connection errors before executing any further code requiring database interaction. This is a common practice in web development to ensure the application functions correctly and securely.
// Connecting to database
$servername = "localhost";
$username = "db_username;
$password = "password";
$dbname = "db_name";
$conn = new mysqli($servername, $username, $password, $dbname);
// Connection error check
if ($conn->connect_error) {
die("Bağlantı hatası: " . $conn->connect_error);
}
// Closing the database connection
$conn->close();
?>
Login.php – Web Authentication
In this code, the user’s inputted username and password are retrieved from the HTML form using the $_POST superglobal variable. The password is also passed through the md5() function to encrypt it for security reasons.
Afterward, an SQL query is executed to check if the entered username and password match the records stored in the MySQL database. The SQL query searches the ‘users’ table in the database for a row matching the entered username and password. The query is executed using the query() method of the MySQLi object.
If the query returns a result with at least one row (i.e., num_rows is greater than 0), the entered username and password are correct, and the user is redirected to the ‘lottery.php’ page. A session is started, and the ‘username’ session variable is set to the entered username using the $_SESSION superglobal variable. A success message is also displayed to the user using the echo statement. The header() function redirects the user to the ‘lottery.php’ page after a delay of two seconds using the ‘Refresh’ HTTP header.
However, if the SQL query returns no results, the entered username and password are incorrect, and an error message is displayed using the echo statement. The button element and the script tag are used to create a button that allows the user to go back to the login page if they entered the wrong credentials.
Overall, this code handles the login process by retrieving user input, checking the database for matching records, and redirecting the user based on the results. It also handles errors by displaying error messages and allowing users to return to the login page if necessary.
// The username and password are being POSTed
$username = $_POST['username'];
$password = md5($_POST['password']);
// The username and password are being checked against the database using an SQL query
$sql = "SELECT * FROM users WHERE username='$username' AND password='$password'";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
// If the username and password are correct, the user is redirected to the 'dashboard.php' page
session_start();
$_SESSION['username'] = $username;
echo '<div class="message">You have successfully logged in.</div>';
header("Refresh: 2; url=dashboard.php");
exit();
} else {
// If the username and password are incorrect, an error message is displayed
echo '<div class="error-message">Incorrect username or password!</div>';
echo '<button onclick="goBack()">Go Back</button>
<script>
function goBack() {
window.history.back();
}
</script>';
}
CSS of login.php
body {
background: linear-gradient(to bottom right, #8E2DE2, #4A00E0, #0000FF);
background-size: cover;
background-attachment: fixed;
color: #fff;
}
.message {
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
padding: 20px;
color: #fff;
font-size: 32px;
font-weight: bold;
text-align: center;
box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.5);
border-radius: 5px;
}
.error-message {
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
padding: 20px;
background-color: #d9534f;
color: #fff;
font-size: 24px;
text-align: center;
}
button {
background-color: #fff;
color: #000;
padding: 10px;
border: none;
border-radius: 5px;
font-size: 16px;
cursor: pointer;
}
button:hover {
background-color: #ddd;
}
Recommended post :Internet of Things (IoT)