Table of Contents
The Caesar Cipher, also known as the shift cipher, is one of the most straightforward and widely known encryption techniques. It is a substitution cipher in which each letter in the plaintext message is shifted a certain number of places down the alphabet. The number of places shifted is the “key” to the cipher.
Julius Caesar used an encryption technique in his messages that the enemies wouldn’t understand, despite the risk of his letters being captured by the enemy. Over time, this method became known as the Caesar Cipher. Today, the Caesar Cipher method is not commonly used because, although it was once secure, the likelihood of the code being cracked is 1 in 25.
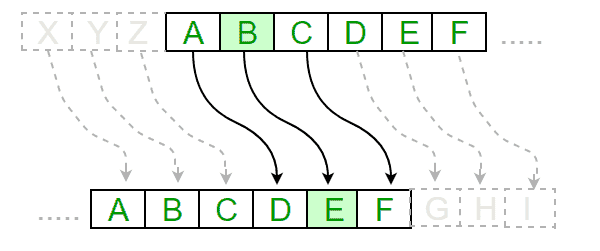
Here’s How Caesar Cipher works?
- Choose a key: The number of positions in each letter in the plaintext will be shifted. For example, if the key is 3, then every letter in the plaintext will be shifted three positions down the alphabet.
- Write the plaintext: Write out the message you want to encrypt.
- Shift the letters: Replace each letter in the plaintext with the letter that is the key number of positions down the alphabet. For example, if the key is 3, A becomes D, B becomes E, C becomes F, and so on.
- Create the ciphertext: The resulting string of letters after the shift is the ciphertext.
Let’s say you want to encode the message “HELLO” with a key of 3.
- Choose a key: In this case, the key is 3.
- Write the plaintext: Write out the message you want to encrypt. In this case, the plaintext is “HELLO.”
- Shift the letters: Replace each letter in the plaintext with the letter that is the key number of positions down the alphabet. For example, if the key is 3, A becomes D, B becomes E, C becomes F, and so on. In this case, the letters in the plaintext are shifted three positions down the alphabet to create the ciphertext. So:
- H becomes K
- E becomes H
- L becomes O
- L becomes O
- O becomes R
So the ciphertext is “KHOOR.”
- Create the ciphertext: The resulting string of letters after the shift is the ciphertext. In this case, the ciphertext is “KHOOR.”
So the encoded message is “KHOOR.” To decode the message, you would shift the letters back by the same number of positions in the opposite direction (in this case, three positions up the alphabet) to reveal the original message.
Recommended Post: Is it safe to use a contactless credit card?
.net with the Caesar Cipher Method
using System;
class CaesarCipher
{
static string Encrypt(string plainText, int key)
{
string result = "";
for (int i = 0; i < plainText.Length; i++)
{
char ch = plainText[i];
if (Char.IsUpper(ch))
{
// Convert uppercase letters to their shifted counterparts
ch = (char)(((int)ch + key - 65) % 26 + 65);
}
else if (Char.IsLower(ch))
{
// Convert lowercase letters to their shifted counterparts
ch = (char)(((int)ch + key - 97) % 26 + 97);
}
result += ch;
}
return result;
}
static void Main(string[] args)
{
string plainText = "HELLO";
int key = 3;
string encryptedText = Encrypt(plainText, key);
Console.WriteLine("Encrypted Text: " + encryptedText);
}
}
This program defines a CaesarCipher class with a static Encrypt method that takes a plain text string and a key (shift) value as inputs and returns the encrypted text as a string. The method loops through each character in the input string and applies the shift based on the character’s ASCII value.
Uppercase and lowercase letters are shifted separately, and non-alphabetic characters are left unchanged. The Main method calls the Encrypt method with the example inputs “HELLO” and three and prints the resulting encrypted text to the console.
Note that this is a very simple implementation of the Caesar Cipher method and should not be used for secure encryption purposes. It is primarily meant for educational purposes.